在Internet上,电子邮件是最广泛使用的一种程序。这样,对于程序员来讲,编写的程序不涉及到电子邮件的处理,现在已经不太可能了。
通常,电子邮件系统用SMTP作为发送协议,用POP3作为邮件接收协议。
下面为编写的一个封装POP3协议的类CPop。
可以复制使用
- /*--------------------------------------------------------------------
- Pop.h : main header file for the POP application
- -----------------------------------------------------------------------*/
- #if !defined(AFX_POP_H__A44B38B6_697C_11D1_881E_00001C302581__INCLUDED_)
- #define AFX_POP_H__A44B38B6_697C_11D1_881E_00001C302581__INCLUDED_
- #define CONNECTION_CHECK 0
- #define USER_CHECK 1
- #define PASSWORD_CHECK 2
- #define QUIT_CHECK 3
- #define DELETE_CHECK 4
- #define RSET_CHECK 5
- #define STAT_CHECK 6
- #define NOOP_CHECK 7
- #define LIST_CHECK 8
- #define RETR_CHECK 9
- /////////////////////////////////////////////////////////////////////////////
- class CPop
- {
- public:
- BOOL List();
- CWordArray m_SizeOfMsg;
- CString GetErrorMessage(); // If there is any error this will return it method
- CString GetPassword(); // Getting Password stored in class
- void SetPassword(CString& Password); // Setting Password in class
- CString GetUser(); // Getting user name stored in class
- void SetUser(CString& User); // Setting user name in class
- CString GetHost(); // Getting Host name (email server name) stored in class
- void SetHost(CString& Host); // Setting Host name (email server name) in class
- BOOL Connect(); // Connecting to email server
- int GetTotalMailSize(); // it returns the Total Mail Size
- int GetNumberOfMails(); // It return the number of mails
- CString GetMsgContents();
- BOOL Statistics(); // issue the STAT command on email server
- BOOL Retrieve(int MsgNumber); // Getting any particular mail message
- BOOL Reset(); // issue the reset command on email server
- int GetMessageSize(int MsgNumber); // Return a size of any particular mail
- BOOL Noop(); // issue the NOOP command on email server
- BOOL Disconnect(); // issue the QUIT command on email server
- BOOL Delete(int& MsgNumber); // Deleteing a particular message from email server
- BOOL Connect(CString& Host, CString& User, CString& Password);
- CPop();
- virtual ~CPop();
- private:
- CString m_ErrorMessage;
- BOOL CheckResponse(int ResponseType);
- CString m_Password;
- CString m_User;
- CString m_Host;
- CString m_MsgContents;
- int m_TotalSize;
- int m_NumberMail;
- CSocket m_PopServer;
- };
- /#endif // !defined(AFX_POP_H__A44B38B6_697C_11D1_881E_00001C302581__INCLUDED_)
-
- /*-----------------------------------------------------------------------------------------------
- // Pop.cpp : Defines the class behaviors for the application.
- ---------------------------------------------------------------------------------------------------*/
- #include "stdafx.h"
- #include "Pop.h"
- #ifdef _DEBUG
- #define new DEBUG_NEW
- #undef THIS_FILE
- static char THIS_FILE[] = __FILE__;
- #endif
- ////////////////////////////////////////////////////////////////////
- // CPop Class
- //////////////////////////////////////////////////////////////////////
- //////////////////////////////////////////////////////////////////////
- // Construction/Destruction
- //////////////////////////////////////////////////////////////////////
- CPop::CPop()
- {
- m_PopServer.Create();
- }
- //{4EEC1C91-6BE1-11d1-8824-00001C302581}
- CPop::~CPop()
- {
- m_PopServer.Close();
- }
- BOOL CPop::Connect(CString & Host, CString & User, CString & Password)
- {
- char buf [512];
- if (!m_PopServer.Connect(Host,110)) // 110 Pop3 Port
- {
- m_ErrorMessage = _T("Server cannot be connected");
- return FALSE;
- }
- else
- {
- if(CheckResponse(CONNECTION_CHECK)==FALSE)
- return FALSE;
- wsprintf (buf, "USER %s\r\n", (LPCSTR) User);
- m_PopServer.Send(buf, strlen (buf));
- if(CheckResponse(USER_CHECK)==FALSE)
- return FALSE;
- wsprintf (buf, "PASS %s\r\n", (LPCSTR) Password);
- m_PopServer.Send(buf, strlen (buf));
- if (CheckResponse(PASSWORD_CHECK)==FALSE)
- return FALSE;
- return TRUE;
- }
- }
- BOOL CPop::Delete(int & MsgNumber)
- {
- char buf [512];
- wsprintf (buf, "DELE %d\r\n",MsgNumber );
- m_PopServer.Send(buf, strlen (buf));
- if (CheckResponse(DELETE_CHECK)==FALSE)
- return FALSE;
- else
- return TRUE;
- }
- BOOL CPop::Disconnect()
- {
- char buf [512];
- wsprintf (buf, "QUIT \r\n");
- m_PopServer.Send(buf, strlen (buf));
- if (CheckResponse(QUIT_CHECK)==FALSE)
- return FALSE;
- else
- return TRUE;
- }
- BOOL CPop::Noop()
- {
- char buf [512];
- wsprintf (buf, "NOOP \r\n");
- m_PopServer.Send(buf, strlen (buf));
- if (CheckResponse(NOOP_CHECK)==FALSE)
- return FALSE;
- else
- return TRUE;
- }
- // Return the Msg Size for given msg number
- int CPop::GetMessageSize(int MsgNumber)
- {
- if(m_SizeOfMsg.GetSize() < MsgNumber+1)
- return 0;
- else
- return m_SizeOfMsg[MsgNumber+1];
- }
- BOOL CPop::Reset()
- {
- char buf [512];
- wsprintf (buf, "RSET \r\n");
- m_PopServer.Send(buf, strlen (buf));
- if (CheckResponse(RSET_CHECK)==FALSE)
- return FALSE;
- else
- return TRUE;
- }
- // MsgContents will hold the msg body
- BOOL CPop::Retrieve(int MsgNumber)
- {
- char buf [512];
- wsprintf (buf, "RETR %d\r\n",MsgNumber );
- m_PopServer.Send(buf, strlen (buf));
- if (CheckResponse(RETR_CHECK)==FALSE)
- return FALSE;
- else
- return TRUE;
- }
- BOOL CPop::Statistics()
- {
- char buf [512];
- wsprintf (buf, "STAT \r\n");
- m_PopServer.Send(buf, strlen (buf));
- if (CheckResponse(STAT_CHECK)==FALSE)
- return FALSE;
- else
- return TRUE;
- }
- CString CPop::GetMsgContents()
- {
- return m_MsgContents;
- }
- int CPop::GetNumberOfMails()
- {
- return m_NumberMail;
- }
- int CPop::GetTotalMailSize()
- {
- return m_TotalSize;
- }
- BOOL CPop::Connect()
- {
- Connect(m_Host, m_User, m_Password);
- return TRUE;
- }
- void CPop::SetHost(CString & Host)
- {
- m_Host = Host;
- }
- CString CPop::GetHost()
- {
- return m_Host;
- }
- void CPop::SetUser(CString & User)
- {
- m_User = User;
- }
- CString CPop::GetUser()
- {
- return m_User;
- }
- void CPop::SetPassword(CString & Password)
- {
- m_Password = Password;
- }
- CString CPop::GetPassword()
- {
- return m_Password;
- }
- BOOL CPop::CheckResponse(int ResponseType)
- {
- char buf [1000];
- for (int i=0;i<512;i++)
- buf[i]='\0';
- m_PopServer.Receive(buf, sizeof(buf));
- switch (ResponseType)
- {
- case CONNECTION_CHECK:
- if (strnicmp(buf,"-ERR", 4) == 0)
- {
- m_ErrorMessage = _T("Bad Connection");
- return FALSE;
- }
- break;
- case USER_CHECK:
- if (strnicmp(buf,"-ERR", 4) == 0)
- {
- m_ErrorMessage = _T("Bad User Name");
- return FALSE;
- }
- break;
- case PASSWORD_CHECK:
- if (strnicmp(buf,"-ERR", 4) == 0)
- {
- m_ErrorMessage = _T("Bad Password Name");
- return FALSE;
- }
- break;
- case QUIT_CHECK:
- if (strnicmp(buf,"-ERR", 4) == 0)
- {
- m_ErrorMessage = _T("Error occured during QUIT");
- return FALSE;
- }
- break;
- case DELETE_CHECK:
- if (strnicmp(buf,"-ERR", 4) == 0)
- {
- m_ErrorMessage = _T("Error occured during DELE");
- return FALSE;
- }
- break;
- case RSET_CHECK:
- if (strnicmp(buf,"-ERR", 4) == 0)
- {
- m_ErrorMessage = _T("Error occured during RSET");
- return FALSE;
- }
- break;
- case STAT_CHECK:
- if (strnicmp(buf,"-ERR", 4) == 0)
- {
- m_ErrorMessage = _T("Error occured during STAT");
- return FALSE;
- }
- else
- {
- BOOL EmailNumber = TRUE;
- for (char *p = buf; *p != '\0'; p++)
- {
- if (*p == '\t' || *p == ' ')
- {
- if(EmailNumber == TRUE)
- {
- m_NumberMail = atoi(p);
- EmailNumber = FALSE;
- }
- else
- {
- m_TotalSize = atoi(p);
- return TRUE;
- }
- }
- }
- }
- break;
- case NOOP_CHECK:
- if (strnicmp(buf,"-ERR", 4) == 0)
- {
- m_ErrorMessage = _T("Error occured during NOOP");
- return FALSE;
- }
- break;
- case LIST_CHECK:
- if (strnicmp(buf,"-ERR", 4) == 0)
- {
- m_ErrorMessage = _T("Error occured during LIST");
- return FALSE;
- }
- else
- {
- m_PopServer.Receive(buf, sizeof(buf));
- for (char *p = buf; *p != '.'; p++)
- if (*p == '\t' || *p == ' ')
- m_SizeOfMsg.Add(atoi(p));
- }
- break;
- case RETR_CHECK:
- if (strnicmp(buf,"-ERR", 4) == 0)
- {
- m_ErrorMessage = _T("Error occured during RETR");
- return FALSE;
- }
- else
- {
- char temp[9000];
- m_PopServer.Receive(temp, sizeof(temp));
- m_MsgContents = temp;
- }
- break;
- }
- return TRUE;
- }
- CString CPop::GetErrorMessage()
- {
- return m_ErrorMessage;
- }
- BOOL CPop::List()
- {
- char buf [512];
- wsprintf (buf, "LIST \r\n");
- m_PopServer.Send(buf, strlen (buf));
- if (CheckResponse(LIST_CHECK)==FALSE)
- return FALSE;
- else
- return TRUE;
- }
复制代码 上位机VC MFC程序开发精典实例大全源码与视频讲解配套下载408例 经历1年的编程与录制点击进入查看
如果您认可,可联系功能定制! 如果您着急,充值会员可直接联系发您资料! 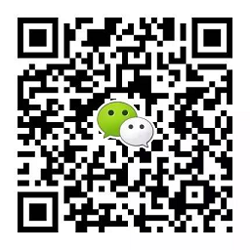
|